5.2. Stacks¶
5.2.1. Objectives¶
Upon completion of this module, students will be able to:
Describe the Stack Abstract Data Type/Data Structure and the characteristics of a Stack
Implement Stacks in Java using an Array-Based or Linked-Chain approach
Develop and use Stack methods
Test the functionality of Stack
Evaluate a range of applications / use cases to determine if use of the Stack Data Structure is appropriate
5.2.1.1. Suggested Reading¶
Chapters 5 - 6 Bags from Data Structures and Abstractions with Java, 4th edition by Frank M. Carrano and Timothy Henry
5.2.2. Interactive: Introduction to Stacks¶
The Stack Interface
package stack;
/**
* An interface for the ADT stack.
*
* @author Frank M. Carrano
* @author Timothy M. Henry
* @author maellis1
* @version May 2020
*/
public interface StackInterface<T> {
/**
* Adds a new entry to the top of this stack.
*
* @param newEntry
* An object to be added to the stack.
*/
public void push(T newEntry);
/**
* Removes and returns this stack's top entry.
*
* @return The object at the top of the stack.
* @throws stack.EmptyStackException
* if the stack is empty before the operation.
*/
public T pop();
/**
* Retrieves this stack's top entry.
*
* @return The object at the top of the stack.
* @throws stack.EmptyStackException
* if the stack is empty.
*/
public T peek();
/**
* Detects whether this stack is empty.
*
* @return True if the stack is empty.
*/
public boolean isEmpty();
/** Removes all entries from this stack. */
public void clear();
} // end StackInterface
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
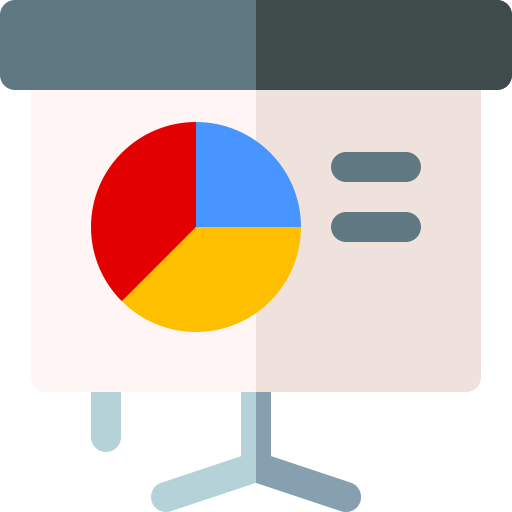
5.2.3. Checkpoint 1¶
5.2.4. Interactive: Stack Memory Example¶
5.2.5. Checkpoint 2¶
5.2.6. Stacks Array-Based Design¶
5.2.7. Checkpoint 3¶
5.2.8. Stacks Array Implementation¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
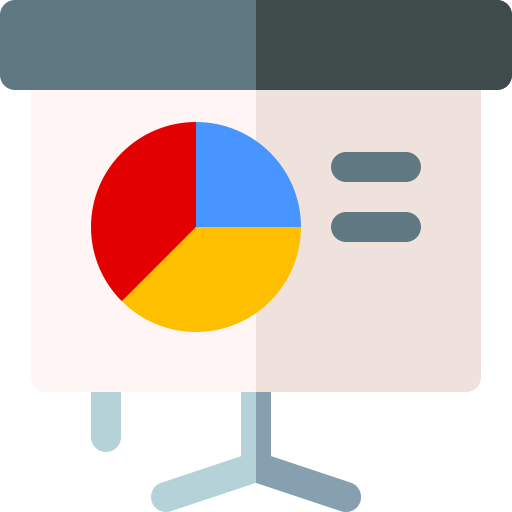
5.2.9. Stacks Linked Chain Implementation¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
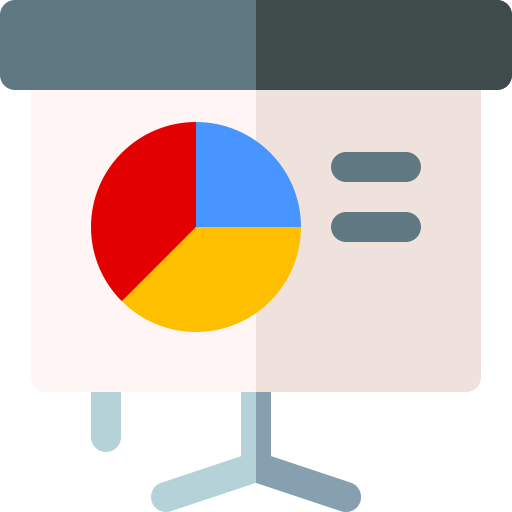
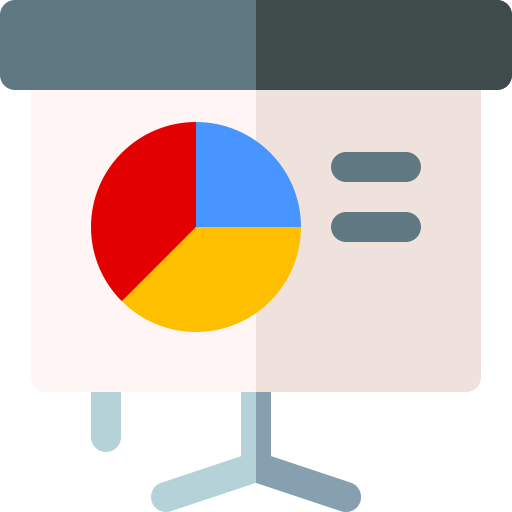