7.3. Recursion¶
7.3.1. Objectives¶
Upon completion of this module, students will be able to:
Trace recursive methods
Implement recursive methods
Consider the efficiency of recursive methods
7.3.1.1. Suggested Reading¶
Chapter 7 from Data Structures and Abstractions with Java, 4th edition by Frank M. Carrano and Timothy Henry
7.3.2. Introduction to Recursion¶
7.3.2.1. Intro to Recursion, Part 1¶
7.3.2.2. Intro to Recursion, Part 2¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
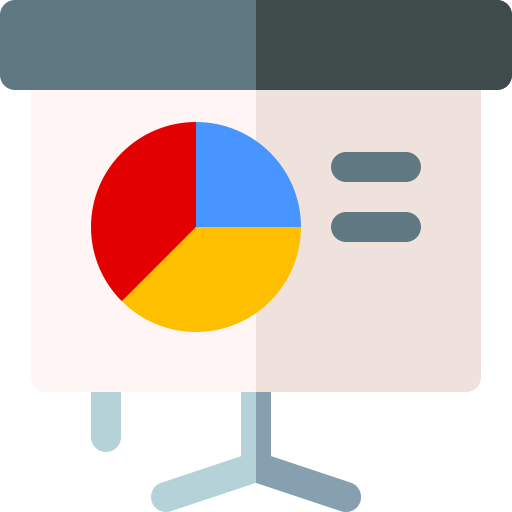
7.3.3. Checkpoint 1¶
7.3.4. Interactive: More Recursion : Factorial Examples¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
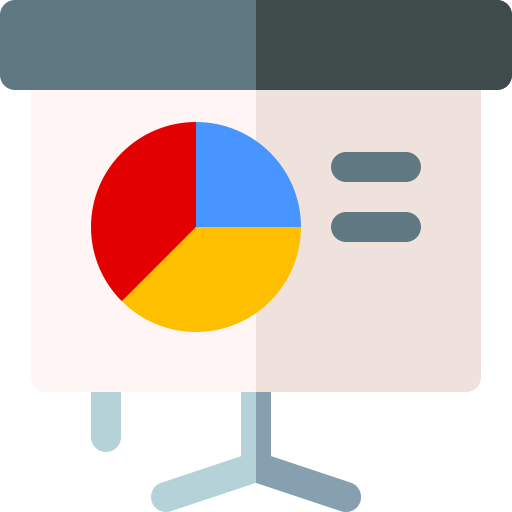
7.3.5. Programming Practice: Recursion 1¶
7.3.6. Interactive: Recursion on Arrays: Display an Array¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
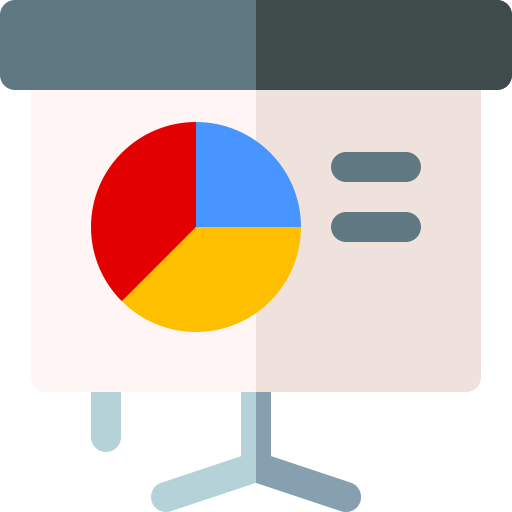
Correction to note!
The code in the second example in this video is missing the {}
in the if
block. It should be:
public static void displayArray2(int[] array, int first, int last)
{
if (first <= last) {
displayArray2(array, first, last - 1);
System.out.print(array[last] + " ");
}
}
7.3.7. Checkpoint 2¶
7.3.8. Interactive: Recursion on Arrays: Display the Middle of an Array¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
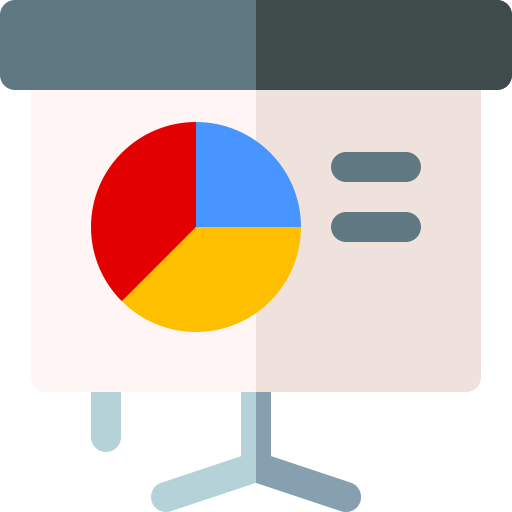
7.3.9. Checkpoint 3¶
7.3.10. Programming Practice: Recursion 2¶
7.3.11. Interactive: Recursion on Linked Chains¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
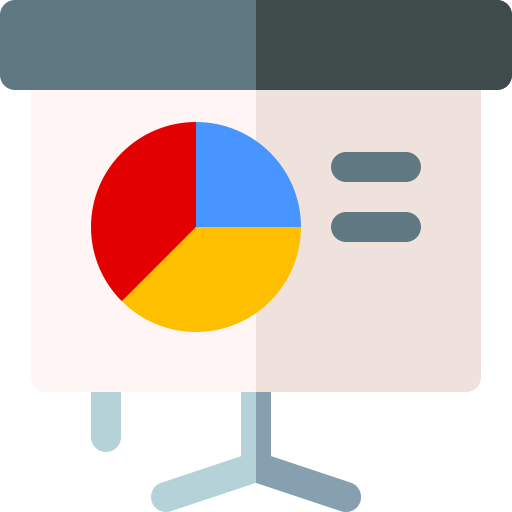
7.3.12. Interactive: Tower of Hanoi¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
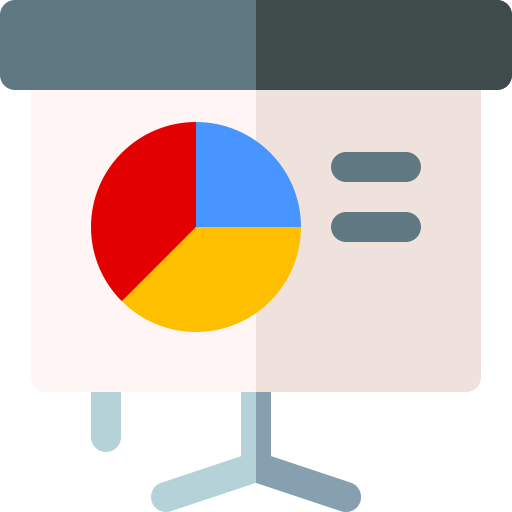
7.3.13. Checkpoint 4¶
7.3.14. Interactive: Recursion Wrap Up¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
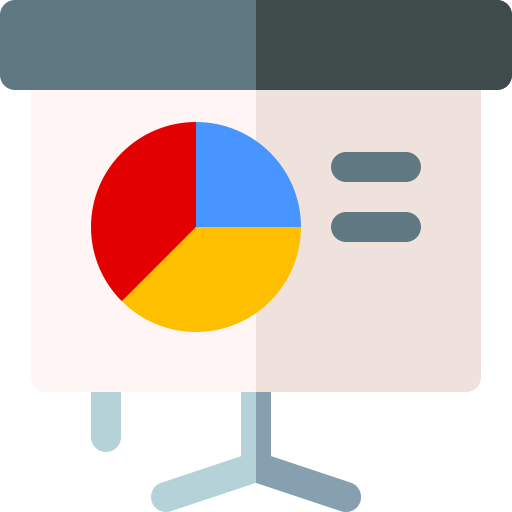