9.1. Lists¶
9.1.1. Overview & Objectives¶
Upon completion of this module, students will be able to:
Distinguish properties and use cases for a list from other ADT(stack, queues, bags)
Implement lists in java using an Array-Based or Linked-Chain approach
Consider various design approaches and corresponding efficiency
Trace and debug list implementations
9.1.1.1. Suggested Reading:¶
Chapters 12 - 14: Lists, A List Implementation That Uses an Array, A List Implementation That Links Data from Data Structures and Abstractions with Java, 4th edition by Frank M. Carrano and Timothy Henry
9.1.2. Introduction to Lists¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
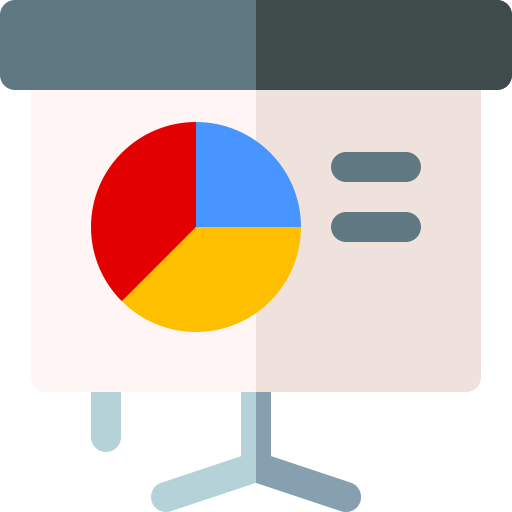
The List Interface
- Download to run and explore the java files (see below) from the video on your own in eclipse. You may download the standalone *.java file for this example. To run the standalone *.java file you will need to
create a new Eclipse project, then
create a package within the project called “example” (the package named at the top of the class MUST match the package the file is placed in within the Eclipse project), and finally
download and import the standalone *.java file(s) to the created package.

package list;
/**
* An interface for the ADT list. Entries in a list have positions that begin
* with 0
*
* @author Frank M. Carrano
* @author Timothy M. Henry
* @author maellis1
* @version Aug 2020
*/
public interface ListInterface {
/**
* Adds a new entry to the end of this list. Entries currently in the list
* are unaffected. The list's size is increased by 1.
*
* @param newEntry
* The object to be added as a new entry.
*/
public void add(T newEntry);
/**
* Adds a new entry at a specified position within this list. Entries
* originally at and above the specified position are at the next higher
* position within the list. The list's size is increased by 1.
*
* @param newPosition
* An integer that specifies the desired position of the new
* entry.
* @param newEntry
* The object to be added as a new entry.
* @throws IndexOutOfBoundsException
* if either newPosition less than 0 or newPosition greater than
* getLength().
*/
public void add(int newPosition, T newEntry);
/**
* Removes the entry at a given position from this list. Entries originally
* at positions higher than the given position are at the next lower
* position within the list, and the list's size is decreased by 1.
*
* @param givenPosition
* An integer that indicates the position of the entry to be
* removed.
* @return A reference to the removed entry.
* @throws IndexOutOfBoundsException
* if either givenPosition less than 0 or givenPosition greater
* than or equal to getLength().
*/
public T remove(int givenPosition);
/** Removes all entries from this list. */
public void clear();
/**
* Replaces the entry at a given position in this list.
*
* @param givenPosition
* An integer that indicates the position of the entry to be
* replaced.
* @param newEntry
* The object that will replace the entry at the position
* givenPosition.
* @return The original entry that was replaced.
* @throws IndexOutOfBoundsException
* if either givenPosition less than 0 or givenPosition greater
* than or equal to getLength().
*/
public T replace(int givenPosition, T newEntry);
/**
* Retrieves the entry at a given position in this list.
*
* @param givenPosition
* An integer that indicates the position of the desired entry.
* @return A reference to the indicated entry.
* @throws IndexOutOfBoundsException
* if either givenPosition less than 0 or givenPosition greater
* than getLength().
*/
public T getEntry(int givenPosition);
/**
* Retrieves all entries that are in this list in the order in which they
* occur in the list.
*
* @return A newly allocated array of all the entries in the list. If the
* list is empty, the returned array is empty.
*/
public Object[] toArray();
/**
* Sees whether this list contains a given entry.
*
* @param anEntry
* The object that is the desired entry.
* @return True if the list contains anEntry, or false if not.
*/
public boolean contains(T anEntry);
/**
* Gets the length of this list.
*
* @return The integer number of entries currently in the list.
*/
public int getLength();
/**
* Sees whether this list is empty.
*
* @return True if the list is empty, or false if not.
*/
public boolean isEmpty();
} // end ListInterface
9.1.3. Checkpoint 1¶
9.1.4. Interactive: LinkedList Add() Implementation¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
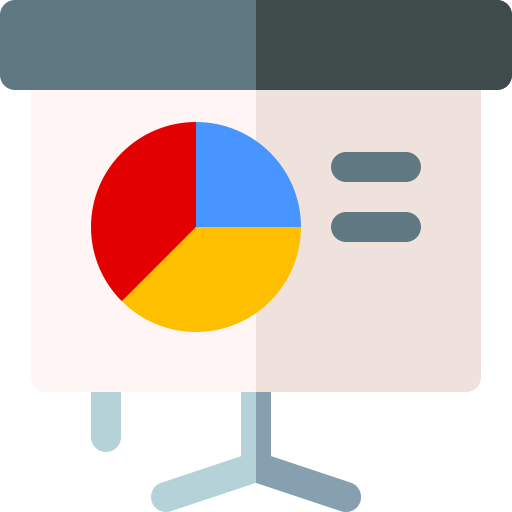
9.1.5. Checkpoint 2¶
9.1.6. Interactive: Tracing Add() with Debugger¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
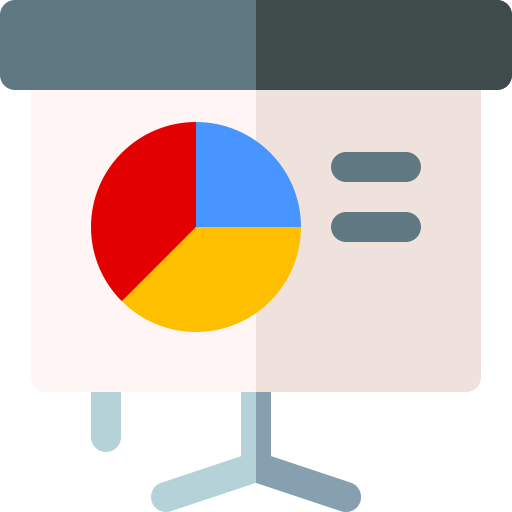
9.1.7. Interactive: LinkedList Remove()¶
Follow Along, Practice and Explore
Download to run and explore the corresponding project from the video on your own in eclipse. The project CS2-Support is required for the sample project above. It is also used in your course projects. To download the CS2-Support you must first complete the configuration steps for your first lab. You will then be able to download it via eclipse using the blue down arrow icon or using the Project Menu and selecting “Download Assignment…”

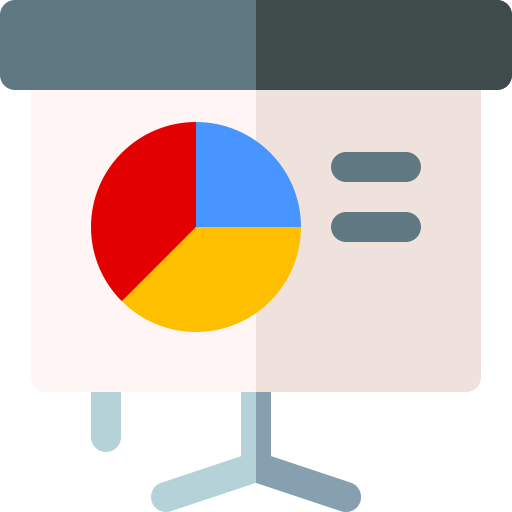
9.1.8. Checkpoint 3¶
9.1.9. Programming Practice: Lists 1¶
9.1.10. Interactive: LinkedList Details and Options¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
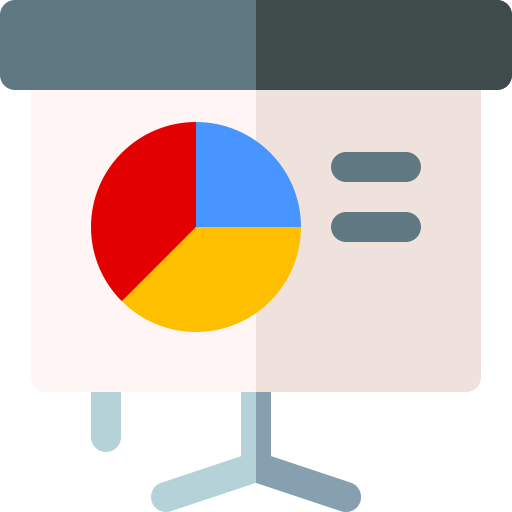
9.1.11. Checkpoint 4¶
9.1.12. Interactive: An Array Implementation of a List¶
Follow Along and Engage
Download the slides corresponding to the video. Take notes on them as you watch the video, practice drawing diagrams yourself!
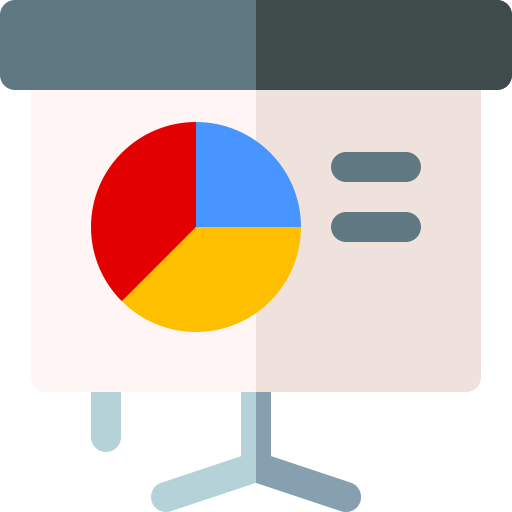
Note: Documentation in the code refers to variable `length` incorrectly, it should be `numberOfEntries